Firebug has a great built in JavaScript performance profiling tool, but it's not supported in IE and sadly that's what the bulk of Internet users browse with. In particular, IE profiling is something of a dark art. There's not a lot of resource available to profile that I could find that was particularly useful.
So I've written a library that allows me to drop in performance timing relatively unobtrusively.
Download time.js (compressed time.js)
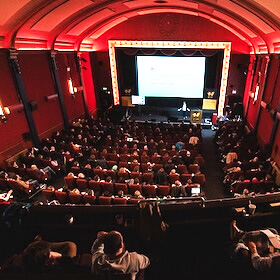
UK EVENTAttend ffconf.org 2024
The conference for people who are passionate about the web. 8 amazing speakers with real human interaction and content you can't just read in a blog post or watch on a tiktok!
£249+VAT - reserve your place today
Essentially this only creating timers where you ask it to. However, the key difference from the usual start/stop function is that you can just point the library at a function and it will time it when it's executed.
Reporting
time.report() time.report(label) time.setReportMethod(function) time.setLineReportMethod(function)
Calling time.report() will show the complete report. If a label is passed in, it will show reports for only that label.
The setter functions allow you to output the reports in your own way, i.e. if you want the output in Firebug's console, or added to a floating DIV you have created. See the performance test example for a working example.
Timing Methods
time.start(label) time.stop(label) time.func([label], function) time.event([label], element/s, event type)
start/stop
The start and stop must have the same label to tie up the timer.
Example
<script src="time.js" type="text/javascript"></script> <script type="text/javascript"> time.start('page load'); window.onload = function() { time.stop('page load'); time.report(); } </script>
func
This can time both static functions or anonymous function. This is where the ease of adding timing comes in.
The key to timing functions, is that the function reference is passed back from time.func() - so you can wrap anonymous function with the timer or you can point event hooks to time static functions.
Static Example
<script src="time.js" type="text/javascript"></script> <script type="text/javascript"> function doJob() { // do some big job } // ... further down the page time.func(doJob); </script>
Anonymous Example
<script src="time.js" type="text/javascript"></script> <script type="text/javascript"> window.onload = time.func('page load', function() { document.getElementById('link').onclick = time.func(function() { // do some job }); }); </script>
event
Time a pre-assigned event on an element. If the event hasn't been assigned yet, the time will not be able to attach to anything.
The event timer also supports attaching to multiple elements at once.
Example
<script src="time.js" type="text/javascript"></script> <script type="text/javascript"> window.onload = function() { var link = document.getElementById('link'); link.onclick = doSomething; // predefined function time.event(link, 'click'); // also supports 'onclick' // alternative version var allLinks = document.getElementsByTagName('a'); time.event(allLinks, 'click'); // hooks all onclick events on links }; </script>
Tools
I've created a little bookmarklet to fire the report whenever you want and a jQuery plugin for the event timer:
Bookmarklet: Report
jQuery plugin: time (note that you still need to include the time.js library)
Usage:
$('a').time('click');